Typescript, undefined and null
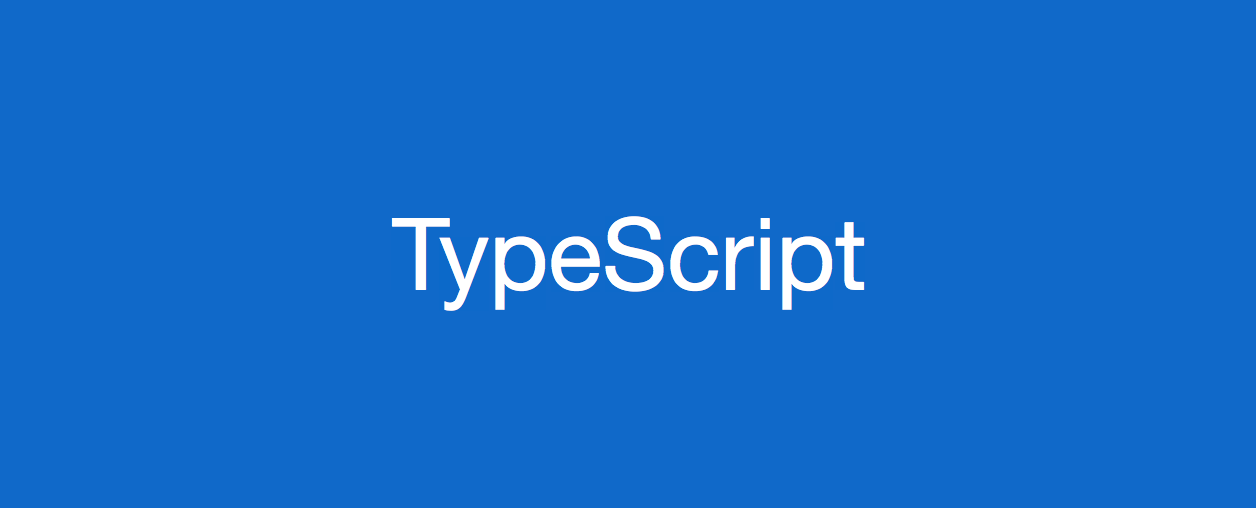
In Typescript, undefined and null are sub-types of any other type (string, boolean, number, void), which means that you can use undefined or null to replace any other type.
With this example:
|
|
So is completely valid to call this function like this:
|
|
But after Typescript 2.0, we can tell Typescript to treat undefined and null like separated types. We can do this by enabling "strictNullChecks": true
in tsconfig.json
.
|
|
With this enabled the sum(undefined, null) will not be valid anymore.
How easy is that :)